id = 123; | l-value | name << endl; | r-value | gpa; | r-value | - The general arrow operator syntax. The left-hand operand is a pointer to a structure object, and the right-hand operator is the name of one of the object's fields.
- Examples illustrate the arrow operator based on the student examples in Figure 3(b).
Choosing the correct selection operator  - Choose the arrow operator if the left-hand operand is a pointer.
- Otherwise, choose the dot operator.
Moving Structures Within A ProgramA basket is a convenient way to carry and move many items by holding its handle. Similarly, a structure object is convenient for a program to "hold" and move many data items with a single (variable) name. Specifically, a program can assign one structure to another (as long as they are instances of the same structure specification), pass them as arguments to functions, and return them from functions as the function's return value. Assignment is a fundamental operation regardless of the kind of data involved. The C++ code to assign one structure to another is simple and should look familiar: - C++ code defining a new structure object and copying an existing object to it by assignment.
- An abstract representation of a new, empty structure.
- An abstract representation of how a program copies the contents of an existing structure to another structure.
Function ArgumentOnce the program creates a structure object, passing it to a function looks and behaves like passing a fundamental-type variable. If we view the function argument as a new, empty structure object, we see that argument passing copies one structure object to another, behaving like an assignment operation. - The first part of the C++ code fragment defines a function (similar to a Java method). The argument is a student structure. The last statement (highlighted) passes a previously created and initialized structure as a function argument.
- In this abstract representation, a program copies the data stored in s2 to the function argument temp . The braces forming the body of the print function create a new scope distinct from the calling scope where the program defines s2 . The italicized variable names label and distinguish the illustrated objects.
Function Return ValueJust as a program can pass a structure into a function as an argument, so it can return a structure as the function's return value, as demonstrated by the following example: - C++ code defining a function named read that returns a structure. The program defines and initializes the structure object, temp , in local or function scope with a series of console read operations and returns it as the function return value. The assignment operator saves the returned structure in s3 .
- A graphical representation of the return process. Returning an object with the return operator copies the local object, temp , to the calling scope, where the program saves it in s1 .
cppreference.comStruct declaration. A struct is a type consisting of a sequence of members whose storage is allocated in an ordered sequence (as opposed to union, which is a type consisting of a sequence of members whose storage overlaps). The type specifier for a struct is identical to the union type specifier except for the keyword used: Syntax Explanation Forward declaration Keywords Notes Example Defect reports References See also |
[ edit ] Syntax | attr-spec-seq (optional) name (optional) struct-declaration-list | (1) | | | attr-spec-seq (optional) name | (2) | | | name | - | the name of the struct that's being defined | struct-declaration-list | - | any number of variable declarations, declarations, and declarations. Members of incomplete type and members of function type are not allowed (except for the flexible array member described below) | attr-spec-seq | - | (C23)optional list of , applied to the struct type | [ edit ] ExplanationWithin a struct object, addresses of its elements (and the addresses of the bit-field allocation units) increase in order in which the members were defined. A pointer to a struct can be cast to a pointer to its first member (or, if the member is a bit-field, to its allocation unit). Likewise, a pointer to the first member of a struct can be cast to a pointer to the enclosing struct. There may be unnamed padding between any two members of a struct or after the last member, but not before the first member. The size of a struct is at least as large as the sum of the sizes of its members. If a struct defines at least one named member, it is allowed to additionally declare its last member with incomplete array type. When an element of the flexible array member is accessed (in an expression that uses operator or with the flexible array member's name as the right-hand-side operand), then the struct behaves as if the array member had the longest size fitting in the memory allocated for this object. If no additional storage was allocated, it behaves as if an array with 1 element, except that the behavior is undefined if that element is accessed or a pointer one past that element is produced. Initialization and the assignment operator ignore the flexible array member. sizeof omits it, but may have more trailing padding than the omission would imply. Structures with flexible array members (or unions who have a recursive-possibly structure member with flexible array member) cannot appear as array elements or as members of other structures. s { int n; double d[]; }; // s.d is a flexible array member struct s t1 = { 0 }; // OK, d is as if double d[1], but UB to access struct s t2 = { 1, { 4.2 } }; // error: initialization ignores flexible array // if sizeof (double) == 8 struct s *s1 = (sizeof (struct s) + 64); // as if d was double d[8] struct s *s2 = (sizeof (struct s) + 40); // as if d was double d[5] s1 = (sizeof (struct s) + 10); // now as if d was double d[1]. Two bytes excess. double *dp = &(s1->d[0]); // OK *dp = 42; // OK s1->d[1]++; // Undefined behavior. 2 excess bytes can't be accessed // as double. s2 = (sizeof (struct s) + 6); // same, but UB to access because 2 bytes are // missing to complete 1 double dp = &(s2->d[0]); // OK, can take address just fine *dp = 42; // undefined behavior *s1 = *s2; // only copies s.n, not any element of s.d // except those caught in sizeof (struct s) | (since C99) | Similar to union, an unnamed member of a struct whose type is a struct without name is known as . Every member of an anonymous struct is considered to be a member of the enclosing struct or union, keeping their structure layout. This applies recursively if the enclosing struct or union is also anonymous. v { union // anonymous union { struct { int i, j; }; // anonymous structure struct { long k, l; } w; }; int m; } v1; v1.i = 2; // valid v1.k = 3; // invalid: inner structure is not anonymous v1.w.k = 5; // valid Similar to union, the behavior of the program is undefined if struct is defined without any named members (including those obtained via anonymous nested structs or unions). | (since C11) | [ edit ] Forward declarationA declaration of the following form | attr-spec-seq (optional) name | | | | hides any previously declared meaning for the name name in the tag name space and declares name as a new struct name in current scope, which will be defined later. Until the definition appears, this struct name has incomplete type . This allows structs that refer to each other: Note that a new struct name may also be introduced just by using a struct tag within another declaration, but if a previously declared struct with the same name exists in the tag name space , the tag would refer to that name [ edit ] Keywords[ edit ] notes. See struct initialization for the rules regarding the initializers for structs. Because members of incomplete type are not allowed, and a struct type is not complete until the end of the definition, a struct cannot have a member of its own type. A pointer to its own type is allowed, and is commonly used to implement nodes in linked lists or trees. Because a struct declaration does not establish scope , nested types, enumerations and enumerators introduced by declarations within struct-declaration-list are visible in the surrounding scope where the struct is defined. [ edit ] ExamplePossible output: [ edit ] Defect reportsThe following behavior-changing defect reports were applied retroactively to previously published C standards. DR | Applied to | Behavior as published | Correct behavior | | C11 | members of anonymous structs/unions were considered members of the enclosing struct/union | they retain their memory layout | [ edit ] References- C23 standard (ISO/IEC 9899:2024):
- 6.7.2.1 Structure and union specifiers (p: TBD)
- C17 standard (ISO/IEC 9899:2018):
- 6.7.2.1 Structure and union specifiers (p: 81-84)
- C11 standard (ISO/IEC 9899:2011):
- 6.7.2.1 Structure and union specifiers (p: 112-117)
- C99 standard (ISO/IEC 9899:1999):
- 6.7.2.1 Structure and union specifiers (p: 101-104)
- C89/C90 standard (ISO/IEC 9899:1990):
- 3.5.2.1 Structure and union specifiers
[ edit ] See also- struct and union member access
- struct initialization
- Recent changes
- Offline version
- What links here
- Related changes
- Upload file
- Special pages
- Printable version
- Permanent link
- Page information
- In other languages
- This page was last modified on 6 January 2024, at 23:45.
- Privacy policy
- About cppreference.com
- Disclaimers
  Structure in C Programming Language with practical examples- Declaring Structure in C
- Variable Declaration with Structure Template
- Variable Declaration after Structure Template
- Initializing by Accessing Member Variables
- Initializing through Initializer List
- Structure as a Member Variable
- Declaring Inside Another Structure
- Structure Pointers
- Passing Structure to a Function
- Run C Programming Online Compiler
It is often convenient to organize related pieces of information together while working with real life entities. In C programming language, structure provides a way to do that by grouping several related variables into one place as structs. Structure may contain different data types such as char, integer, float and group them together to create a user-defined data type. ¶ Declaring Structure in CTo declare a structure, we use the keyword struct followed by an identifier as the structure name. Inside the curly braces we list the member variables with their respective data types. Here, we are creating a structure for devices that contains information about the device’s name, price, and quantity. Notice we put a semicolon after the curly braces unlike the function declaration we have seen previously. ¶ Declaring Structure VariableA structure is a kind of blueprint or template in which we get to decide what types of data we want to keep. To use it, we need to create an instance (variable) of it. Let’s create two structure variables of Device type. We can do that in the following ways, ¶ Variable Declaration with Structure TemplateWe can create instances of the structure with the template in the following way. ¶ Variable Declaration after Structure TemplateWe can also create instances of the structure after the template in the following way Notice that we use the keyword struct followed by the name of the structure we want to create an instance of, and then the variable names. Structure also allows to create array of structure like other data types with the similar syntax. ¶ Initializing Structure VariableSince a structure is just a blueprint not an actual data placeholder, it doesn’t occupy any memory space when declared until an instance is created. Also, structures do not support constructors or functions inside their body. Therefore, we can not initialize member variables within the curly braces. However, we can initialize or assign values to member variables in the following ways: ¶ Initializing by Accessing Member VariablesTo assign or access the value of the member variables of an instance we can access them using dot (.) operator then assign value using assignment operator (=) Notice that we assigned name variable differently from others because, C string is an array of characters which does not support using assignment operator directly. That’s why we used the function strcpy() from string.h header file to achieve the same goal. ¶ Initializing through Initializer ListWe could achieve the same result when declaring an instance of the structure through an initializer list in the following way, ¶ Nested StructuresWhen a structure is used as a member variable of another structure or declared inside another structure, it is referred to as the nesting of structures. Nesting of a structure can be done in the following two ways, ¶ Structure as a Member VariableWe can use a structure as a member variable of another structure as well. We have to declare the structure of the member variable before the structure in which we are nesting our structure. We have nested our structure Model as a member variable inside the structure Device . We can access and initialize the members of the both structures Device and Model in the same way we have seen before. ¶ Declaring Inside Another StructureWe can also declare a structure inside the body of another structure. We can access and assign member variables in the same way we have seen before. ¶ Structure PointersSimilar to other data types, we can use a pointer to refer to the memory address of a structure. To access the member variables, the arrow operator (->) is used with the structure pointer. We can also use pointers as member variables. Sometimes, it is useful to have a pointer member that points to another instance of the same structure, creating a self-referential structure . Consider a scenario where we want to manage desktop information along with the GPU details used in that desktop. We can define a Device structure with an additional pointer member that points to another instance of the same Device structure. In this example, both the desktop and GPU are instances of the Device structure, and a desktop Device structure can point to another Device structure, representing the associated GPU, through a self-referential pointer . ¶ Passing Structure to a Functionwe can pass a structure to a function by the structure itself (pass by value) or a reference to the structure (pass by reference). When we pass by the reference we can modify the actual instance members from inside the function. In the C programming language, directly returning multiple values from a function is not allowed. One workaround is to return a structure from a function. ¶ Run C Programming Online CompilerTo make your learning more effective, exercise the coding examples in the text editor below. Run C programming online All Tutorials in this playlist What is C Programming and How to Start C Programming Getting Started Your First Coding With C Programming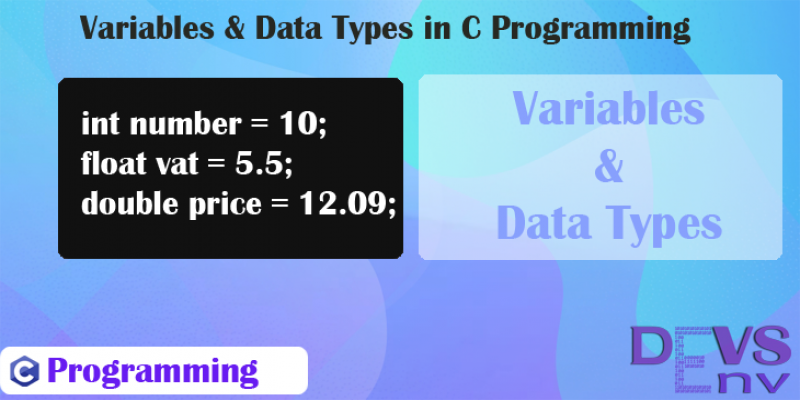 Variable and Data Types with Practical Examples C Programming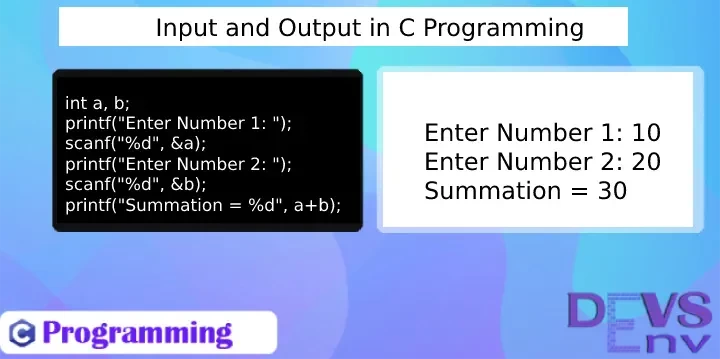 Input and Output in C Programming Conditional in C Programming - if-else, elseif, switch-case Loops in C Programming - for loop, while loop and do-while loop Functions in C Programming - with Practical examples Arrays in C Programming - Algorithm and Practical examples 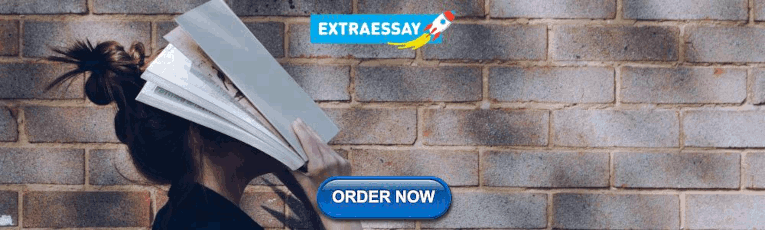 Recursion in C Programming Language with Practical Examples File Handling in C Programming Language with Practical Examples Pointer in C Programming Language with Practical Examples Student Management Complete Application Using C Programming C Project - Complete Calculator Application using C ProgrammingPopular tutorials, vue js 3 - complete advance crud example with vuex, vue-validate, pagination, searching and everything, laravel eloquent vs db query builder [performance and other statistics], laravel role permission management system full example with source code, start wordpress plugin development with react js easily in just few steps, laravel membership management full project demo with source codes, numerical methods for engineers ebook & solution download - download pdf, connect your react app with websocket to get real time data, laravel ecommerce complete web application with admin panel, #ajax with laravel api and more - learn laravel beyond the limit, what is seeder in laravel and why we need it. - Artificial Intelligence (AI)
- Bash Scripting
Bootstrap CSS - Code Editor
- Computer Engineering
- Data Structure and Algorithm
Design Pattern in PHP Design Patterns - Clean Code Interview Prepration Java Programming Laravel PHP Framework Online Business React Native Rust Programming Tailwind CSS Uncategorized Windows Operating system WordPress Development - C-sharp programming
- Design pattern
- Mathematics
- Rust Programming Language
- Windows terminal
- WordPress Plugin Development
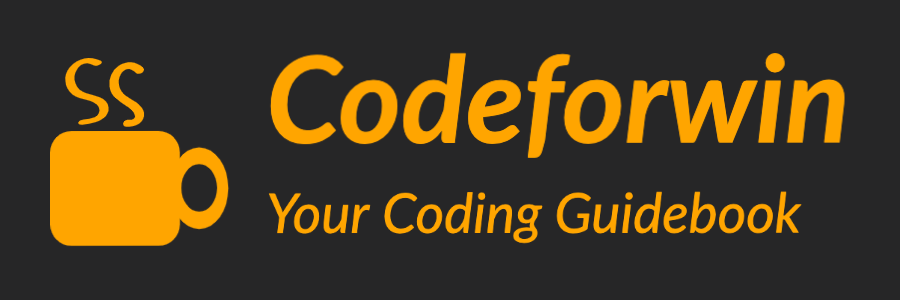 Structures in C programming, need and use- Need of structures?
- What is structure?
- How to declare and define?
- How to create structure object?
- How to access structure members?
- Example program
Structures in C, is an advance and most popular topic in C language. It facilitates you to design your custom data type . In this tutorial, we will learn about structures in C its need, how to declare, define and access structures. Need of structures in C?C has built in primitive and derrived data types . Still not all real world problems can be solved using those types. You need custom data type for different situations. For example, if you need to store 100 student record that consist of name, age and mobile number. To code that you will create 3 array variables each of size 100 i.e. name[100] , age[100] , mobile[100] . For three fields in student record it say seem feasible to you. But, think how cumbersome it would be to manage student record with more than 10 fields, in separate variables for single student. To overcome this we need a user defined data type. In this tutorial I am going to explain how easily we will deal with these situations using structures in C programming language. What is structure in C?Structure is a user defined data type. It is a collection of different data type, to create a new data type. For example, You can define your custom type for storing student record containing name, age and mobile. Creating structure type will allow you to handle all properties (fields) of student with single variable, instead of handling it separately. How to declare, define and access structure members?To declare or define a structure, we use struct keyword. It is a reserved word in the C compiler. You must only use it for structure or its object declaration or definition. Syntax to define a structureHere, structure_name is name of our custom type. memberN_declaration is structure member i.e. variable declaration that structure will have. Example to define a structureLet us use our student example and define a structure to store student object. Points to remember while structure definition- You must terminate structure definition with semicolon ; .
For example, following is an invalid structure definition. - You can define a structure anywhere like global scope (accessible by all functions) or local scope (accessible by particular function).
- Structure member definition may contain other structure type.
How to create structure object (structure variable)?A data type is useless without variables. A data type defines various properties about data stored in memory. To use any type we must declare its variable. Hence, let us learn how to create our custom structure type objects also known as structure variable . In C programming, there are two ways to declare a structure variable: - Along with structure definition
- After structure definition
Declaration along with the structure definitionOut of two ways to declare structure variable. You can declare a structure variable along with structure before terminating the structure definition. So, if you want to declare student type object along with student structure definition you can use this approach. Declaration after structure definitionThe other way to declare, gives you luxury to declare structure variable anywhere in program based on the structure scope. If structure is defined in global scope, we can declare its variable in main() function , any other functions and in the global section too. For above example, if we want to declare its variable with name student1 , it will be declared as given below: How to access structure members (data)?You created structure and its variable. But since structure is a complex data type, you cannot assign any value directly to it using assignment operator . You must assign data to individual structure members separately. C supports two operators to access structure members, using a structure variable. - Dot/period operator .
- Arrow operator ->
Dot/period operator (.) in CDot/period operator also known as member access operator. We use dot operator to access members of simple structure variable. Read more about other operators in C programming language . Arrow operator (->) in CSince structure is a user defined type and you can have pointers to any type. Hence, you may also create pointers to structure. In C language it is illegal to access a structure member from a pointer to structure variable using dot operator. We use arrow operator -> to access structure member from pointer to structure. Example program to demonstrate declare, define and access structure membersIn this example, we will declare a structure type, create structure object and access structure members. I will show how to use both dot and arrow operator to access structure members. Happy coding 😉 - C Data Types
- C Operators
- C Input and Output
- C Control Flow
- C Functions
- C Preprocessors
- C File Handling
- C Cheatsheet
- C Interview Questions
Nested Structure in C with ExamplesPre-requisite: Structures in C A nested structure in C is a structure within structure. One structure can be declared inside another structure in the same way structure members are declared inside a structure. struct name_1 { member1; member2; . . membern; struct name_2 { member_1; member_2; . . member_n; }, var1 } var2; The member of a nested structure can be accessed using the following syntax: Variable name of Outer_Structure.Variable name of Nested_Structure.data member to access - Consider there are two structures Employee (depended structure) and another structure called Organisation(Outer structure) .
- The structure Organisation has the data members like organisation_name,organisation_number.
- The Employee structure is nested inside the structure Organisation and it has the data members like employee_id, name, salary.
For accessing the members of Organisation and Employee following syntax will be used: org.emp.employee_id; org.emp.name; org.emp.salary; org.organisation_name; org.organisation_number; Here, org is the structure variable of the outer structure Organisation and emp is the structure variable of the inner structure Employee. Different ways of nesting structure The structure can be nested in the following different ways: - By separate nested structure
- By embedded nested structure .
1. By separate nested structure: In this method, the two structures are created, but the dependent structure(Employee) should be used inside the main structure(Organisation) as a member. Below is the C program to implement the approach: The size of structure organisation : 68 Organisation Name : GeeksforGeeks Organisation Number : GFG123768 Employee id : 101 Employee name : Robert Employee Salary : 400000 2. By Embedded nested structure: Using this method, allows to declare structure inside a structure and it requires fewer lines of code. Case 1: Error will occur if the structure is present but the structure variable is missing. 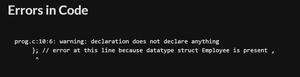 Whenever an embedded nested structure is created, the variable declaration is compulsory at the end of the inner structure, which acts as a member of the outer structure. It is compulsory that the structure variable is created at the end of the inner structure. Case 2: When the structure variable of the inner structure is declared at the end of the inner structure. Below is the C program to implement this approach: Drawback of Nested Structure The drawback in nested structures are: - Independent existence not possible: It is important to note that structure Employee doesn’t exist on its own. One can’t declare structure variable of type struct Employee anywhere else in the program.
- Cannot be used in multiple data structures: The nested structure cannot be used in multiple structures due to the limitation of declaring structure variables within the main structure. So, the most recommended way is to use a separate structure and it can be used in multiple data structures
C Nested Structure Example Below is another example of a C nested structure. College name : GeeksforGeeks Ranking : 7 Student id : 111 Student name : Paul Roll no : 278 Nesting of structure within itself is not allowed. struct student { char name[50]; char address[100]; int roll_no; struct student geek; // Invalid } Passing nested structure to function A nested structure can be passed into the function in two ways: - Pass the nested structure variable at once.
- Pass the nested structure members as an argument into the function.
Let’s discuss each of these ways in detail. 1. Pass the nested structure variable at once: Just like other variables, a nested structure variable can also be passed to the function. Below is the C program to implement this concept: Printing the Details : Organisation Name : GeeksforGeeks Organisation Number : GFG111 Employee id : 278 Employee name : Paul Employee Salary : 5000 2. Pass the nested structure members as arguments into the function: Consider the following example to pass the structure member of the employee to a function display() which is used to display the details of an employee. Accessing Nested Structure Nested Structure can be accessed in two ways: - Using Normal variable.
- Using Pointer variable.
Let’s discuss each of these methods in detail. 1. Using Normal variable: Outer and inner structure variables are declared as normal variables and the data members of the outer structure are accessed using a single dot(.) and the data members of the inner structure are accessed using the two dots. Below is the C program to implement this concept: College ID : 14567 College Name : GeeksforGeeks Student ID : 12 Student Name : Kathy Student CGPA : 7.800000 2. Using Pointer variable: One normal variable and one pointer variable of the structure are declared to explain the difference between the two. In the case of the pointer variable, a combination of dot(.) and arrow(->) will be used to access the data members. Below is the C program to implement the above approach: A nested structure will allow the creation of complex data types according to the requirements of the program. 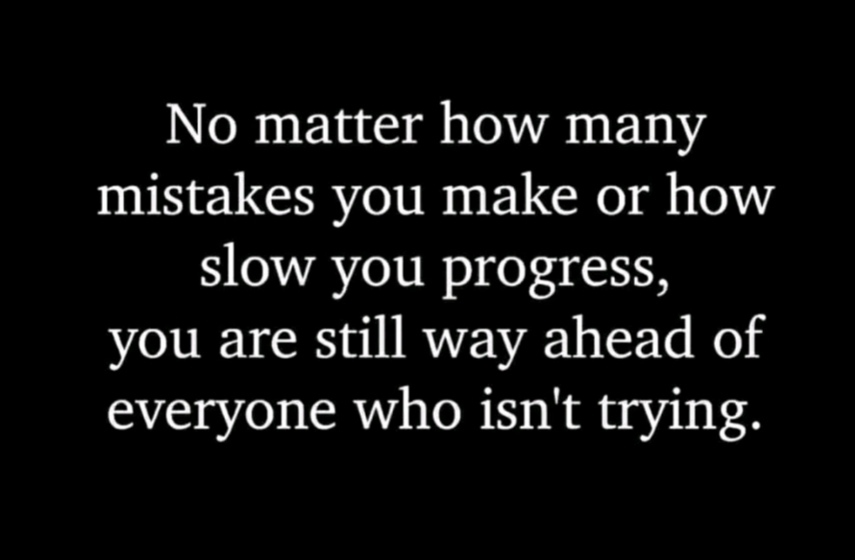 Please Login to comment...Similar reads. - C-Structure & Union
- OpenAI o1 AI Model Launched: Explore o1-Preview, o1-Mini, Pricing & Comparison
- How to Merge Cells in Google Sheets: Step by Step Guide
- How to Lock Cells in Google Sheets : Step by Step Guide
- PS5 Pro Launched: Controller, Price, Specs & Features, How to Pre-Order, and More
- #geekstreak2024 – 21 Days POTD Challenge Powered By Deutsche Bank
Improve your Coding Skills with Practice What kind of Experience do you want to share? - C Programming Tutorial
- Basics of C
- C - Overview
- C - Features
- C - History
- C - Environment Setup
- C - Program Structure
- C - Hello World
- C - Compilation Process
- C - Comments
- C - Keywords
- C - Identifiers
- C - User Input
- C - Basic Syntax
- C - Data Types
- C - Variables
- C - Integer Promotions
- C - Type Conversion
- C - Type Casting
- C - Booleans
- Constants and Literals in C
- C - Constants
- C - Literals
- C - Escape sequences
- C - Format Specifiers
- Operators in C
- C - Operators
- C - Arithmetic Operators
- C - Relational Operators
- C - Logical Operators
- C - Bitwise Operators
- C - Assignment Operators
- C - Unary Operators
- C - Increment and Decrement Operators
- C - Ternary Operator
- C - sizeof Operator
- C - Operator Precedence
- C - Misc Operators
- Decision Making in C
- C - Decision Making
- C - if statement
- C - if...else statement
- C - nested if statements
- C - switch statement
- C - nested switch statements
- C - While loop
- C - For loop
- C - Do...while loop
- C - Nested loop
- C - Infinite loop
- C - Break Statement
- C - Continue Statement
- C - goto Statement
- Functions in C
- C - Functions
- C - Main Function
- C - Function call by Value
- C - Function call by reference
- C - Nested Functions
- C - Variadic Functions
- C - User-Defined Functions
- C - Callback Function
- C - Return Statement
- C - Recursion
- Scope Rules in C
- C - Scope Rules
- C - Static Variables
- C - Global Variables
- Arrays in C
- C - Properties of Array
- C - Multi-Dimensional Arrays
- C - Passing Arrays to Function
- C - Return Array from Function
- C - Variable Length Arrays
- Pointers in C
- C - Pointers
- C - Pointers and Arrays
- C - Applications of Pointers
- C - Pointer Arithmetics
- C - Array of Pointers
- C - Pointer to Pointer
- C - Passing Pointers to Functions
- C - Return Pointer from Functions
- C - Function Pointers
- C - Pointer to an Array
- C - Pointers to Structures
- C - Chain of Pointers
- C - Pointer vs Array
- C - Character Pointers and Functions
- C - NULL Pointer
- C - void Pointer
- C - Dangling Pointers
- C - Dereference Pointer
- C - Near, Far and Huge Pointers
- C - Initialization of Pointer Arrays
- C - Pointers vs. Multi-dimensional Arrays
- Strings in C
- C - Strings
- C - Array of Strings
- C - Special Characters
- C Structures and Unions
- C - Structures
- C - Structures and Functions
- C - Arrays of Structures
- C - Self-Referential Structures
- C - Lookup Tables
- C - Dot (.) Operator
- C - Enumeration (or enum)
- C - Structure Padding and Packing
- C - Nested Structures
- C - Anonymous Structure and Union
- C - Bit Fields
- C - Typedef
- File Handling in C
- C - Input & Output
- C - File I/O (File Handling)
- C Preprocessors
- C - Preprocessors
- C - Pragmas
- C - Preprocessor Operators
- C - Header Files
- Memory Management in C
- C - Memory Management
- C - Memory Address
- C - Storage Classes
- Miscellaneous Topics
- C - Error Handling
- C - Variable Arguments
- C - Command Execution
- C - Math Functions
- C - Static Keyword
- C - Random Number Generation
- C - Command Line Arguments
- C Programming Resources
- C - Questions & Answers
- C - Quick Guide
- C - Cheat Sheet
- C - Useful Resources
- C - Discussion
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
Structures in CA structure in C is a derived or user-defined data type. We use the keyword struct to define a custom data type that groups together the elements of different types. The difference between an array and a structure is that an array is a homogenous collection of similar types, whereas a structure can have elements of different types stored adjacently and identified by a name. We are often required to work with values of different data types having certain relationships among them. For example, a book is described by its title (string), author (string), price (double), number of pages (integer), etc. Instead of using four different variables, these values can be stored in a single struct variable. Declare (Create) a StructureYou can create (declare) a structure by using the "struct" keyword followed by the structure_tag (structure name) and declare all of the members of the structure inside the curly braces along with their data types. To define a structure, you must use the struct statement. The struct statement defines a new data type, with more than one member. Syntax of Structure DeclarationThe format (syntax) to declare a structure is as follows − The structure tag is optional and each member definition is a normal variable definition, such as "int i;" or "float f;" or any other valid variable definition. At the end of the structure's definition, before the final semicolon, you can specify one or more structure variables but it is optional . In the following example we are declaring a structure for Book to store the details of a Book − Here, we declared the structure variable book1 at the end of the structure definition. However, you can do it separately in a different statement. Structure Variable DeclarationTo access and manipulate the members of the structure, you need to declare its variable first. To declare a structure variable, write the structure name along with the "struct" keyword followed by the name of the structure variable. This structure variable will be used to access and manipulate the structure members. The following statement demonstrates how to declare (create) a structure variable Usually, a structure is declared before the first function is defined in the program, after the include statements. That way, the derived type can be used for declaring its variable inside any function. Structure InitializationThe initialization of a struct variable is done by placing the value of each element inside curly brackets. The following statement demonstrates the initialization of structure Accessing the Structure MembersTo access the members of a structure, first, you need to declare a structure variable and then use the dot (.) operator along with the structure variable. The four elements of the struct variable book1 are accessed with the dot (.) operator . Hence, "book1.title" refers to the title element, "book1.author" is the author name, "book1.price" is the price, "book1.pages" is the fourth element (number of pages). Take a look at the following example − Run the code and check its output − In the above program, we will make a small modification. Here, we will put the type definition and the variable declaration together, like this − Note that if you a declare a struct variable in this way, then you cannot initialize it with curly brackets. Instead, the elements need to be assigned individually. When you execute this code, it will produce the following output − Copying StructuresThe assignment (=) operator can be used to copy a structure directly. You can also use the assignment operator (=) to assign the value of the member of one structure to another. Let's have two struct book variables, book1 and book2 . The variable book1 is initialized with declaration, and we wish to assign the same values of its elements to that of book2 . We can assign individual elements as follows − Note the use of strcpy() function to assign the value to a string variable instead of using the "= operator". You can also assign book1 to book2 so that all the elements of book1 are respectively assigned to the elements of book2. Take a look at the following program code − Structures as Function ArgumentsYou can pass a structure as a function argument in the same way as you pass any other variable or pointer. Take a look at the following program code. It demonstrates how you can pass a structure as a function argument − When the above code is compiled and executed, it produces the following result − Pointers to StructuresYou can define pointers to structures in the same way as you define pointers to any other variable. Declaration of Pointer to a StructureYou can declare a pointer to a structure (or structure pointer) as follows − Initialization of Pointer to a StructureYou can store the address of a structure variable in the above pointer variable struct_pointer . To find the address of a structure variable, place the '&' operator before the structure's name as follows − Let's store the address of a struct variable in a struct pointer variable. Accessing Members Using Pointer to a StructureTo access the members of a structure using a pointer to that structure, you must use the → operator as follows − C defines the → symbol to be used with struct pointer as the indirection operator (also called struct dereference operator ). It helps to access the elements of the struct variable to which the pointer reference to. In this example, strptr is a pointer to struct book book1 variable. Hence, strrptr→title returns the title, just like book1.title does. When you run this code, it will produce the following output − Note: The dot (.) operator is used to access the struct elements via the struct variable. To access the elements via its pointer, we must use the indirection (->) operator A struct variable is like a normal variable of primary type, in the sense that you can have an array of struct, you can pass the struct variable to a function, as well as return a struct from a function. You may have noted that you need to prefix "struct type" to the name of the variable or pointer at the time of declaration. This can be avoided by creating a shorthand notation with the help of typedef keyword, which we will explain in a subsequent chapter. Structures are used in different applications such as databases, file management applications, and for handling complex data structures such as tree and linked lists. Bit Fields allow the packing of data in a structure. This is especially useful when memory or data storage is at a premium. Typical examples include − - Packing several objects into a machine word, for example, 1-bit flags can be compacted.
- Reading external file formats − non-standard file formats could be read in, for example, 9-bit integers.
DeclarationC allows us to do this in a structure definition by putting :bit length after the variable. For example − Here, the packed_struct contains 6 members: Four 1 bit flags f1..f3, a 4-bit type and a 9-bit my_int. C automatically packs the above bit fields as compactly as possible, provided that the maximum length of the field is less than or equal to the integer word length of the computer. If this is not the case, then some compilers may allow memory overlap for the fields while others would store the next field in the next word. Learn C practically and Get Certified . Popular TutorialsPopular examples, reference materials, learn c interactively, c introduction. - Getting Started with C
- Your First C Program
C Fundamentals- C Variables, Constants and Literals
- C Data Types
- C Input Output (I/O)
- C Programming Operators
C Flow Control- C if...else Statement
- C while and do...while Loop
- C break and continue
- C switch Statement
- C goto Statement
- C Functions
- C User-defined functions
- Types of User-defined Functions in C Programming
- C Recursion
- C Storage Class
C Programming Arrays- C Multidimensional Arrays
- Pass arrays to a function in C
C Programming Pointers- Relationship Between Arrays and Pointers
- C Pass Addresses and Pointers
- C Dynamic Memory Allocation
- C Array and Pointer Examples
- C Programming Strings
- String Manipulations In C Programming Using Library Functions
- String Examples in C Programming
C Structure and UnionC structs and Pointers C Structure and Function C Programming FilesC Files Examples C Additional Topics- C Keywords and Identifiers
- C Precedence And Associativity Of Operators
- C Bitwise Operators
- C Preprocessor and Macros
- C Standard Library Functions
C Tutorials- Store Information of Students Using Structure
- Add Two Distances (in inch-feet system) using Structures
- Store Data in Structures Dynamically
- Store Information of a Student Using Structure
C Struct ExamplesA structure is a collection of variables of different data types. You will find examples related to structures in this article. To understand examples in this page, you should have the knowledge of the following topics. - C structs and pointers
- C structs and functions
C struct ExamplesSorry about that. Our premium learning platform, created with over a decade of experience and thousands of feedbacks . Learn and improve your coding skills like never before. - Interactive Courses
- Certificates
- 2000+ Challenges
Related TutorialsA man is valued by his works, not his words!Structure assignment and its pitfall in c language. Jan 28 th , 2013 9:47 pm There is a structure type defined as below: 2 3 4 5 | struct __map_t { int code; char name[NAME_SIZE]; char *alias; }map_t; |
If we want to assign map_t type variable struct2 to sturct1 , we usually have below 3 ways: 2 3 4 5 6 7 8 9 10 | struct1.code = struct2.code; strncpy(struct1.name, struct2.name, NAME_SIZE); struct1.alias = struct2.alias; /* Way #2: memcpy the whole memory content of struct2 to struct1 */ memcpy(&struct1, &struct2, sizeof(struct1)); /* Way #3: straight assignment with '=' */ struct1 = struct2; |
Consider above ways, most of programmer won’t use way #1, since it’s so stupid ways compare to other twos, only if we are defining an structure assignment function. So, what’s the difference between way #2 and way #3? And what’s the pitfall of the structure assignment once there is array or pointer member existed? Coming sections maybe helpful for your understanding. The difference between ‘=’ straight assignment and memcpyThe struct1=struct2; notation is not only more concise , but also shorter and leaves more optimization opportunities to the compiler . The semantic meaning of = is an assignment, while memcpy just copies memory. That’s a huge difference in readability as well, although memcpy does the same in this case. Copying by straight assignment is probably best, since it’s shorter, easier to read, and has a higher level of abstraction. Instead of saying (to the human reader of the code) “copy these bits from here to there”, and requiring the reader to think about the size argument to the copy, you’re just doing a straight assignment (“copy this value from here to here”). There can be no hesitation about whether or not the size is correct. Consider that, above source code also has pitfall about the pointer alias, it will lead dangling pointer problem ( It will be introduced below section ). If we use straight structure assignment ‘=’ in C++, we can consider to overload the operator= function , that can dissolve the problem, and the structure assignment usage does not need to do any changes, but structure memcpy does not have such opportunity. The pitfall of structure assignment:Beware though, that copying structs that contain pointers to heap-allocated memory can be a bit dangerous, since by doing so you’re aliasing the pointer, and typically making it ambiguous who owns the pointer after the copying operation. If the structures are of compatible types, yes, you can, with something like: | (dest_struct, source_struct, sizeof(dest_struct)); |
} The only thing you need to be aware of is that this is a shallow copy. In other words, if you have a char * pointing to a specific string, both structures will point to the same string. And changing the contents of one of those string fields (the data that the char points to, not the char itself) will change the other as well. For these situations a “deep copy” is really the only choice, and that needs to go in a function. If you want a easy copy without having to manually do each field but with the added bonus of non-shallow string copies, use strdup: 2 | (dest_struct, source_struct, sizeof (dest_struct)); dest_struct->strptr = strdup(source_struct->strptr); |
This will copy the entire contents of the structure, then deep-copy the string, effectively giving a separate string to each structure. And, if your C implementation doesn’t have a strdup (it’s not part of the ISO standard), you have to allocate new memory for dest_struct pointer member, and copy the data to memory address. Example of trap: 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 | #include <stdlib.h> #include <string.h> #define NAME_SIZE 16 typedef struct _map_t { int code; char name[NAME_SIZE]; char *alias; } map_t; int main() { map_t a, b, c; /* initialize the a's members value */ a.code = 1024; snprintf(a.name, NAME_SIZE, "Controller SW3"); char *alias = "RNC&IPA"; a.alias = alias; /* assign the value via memcpy */ memcpy(&b, &a, sizeof(b)); /* assign the value via '=' */ c = a; return 0; } |
Below diagram illustrates above source memory layout, if there is a pointer field member, either the straight assignment or memcpy , that will be alias of pointer to point same address. For example, b.alias and c.alias both points to address of a.alias . Once one of them free the pointed address, it will cause another pointer as dangling pointer. It’s dangerous!! - Recommend use straight assignment ‘=’ instead of memcpy.
- If structure has pointer or array member, please consider the pointer alias problem, it will lead dangling pointer once incorrect use. Better way is implement structure assignment function in C, and overload the operator= function in C++.
- stackoverflow.com: structure assignment or memcpy
- stackoverflow.com: assign one struct to another in C
- bytes.com: structures assignment
- wikipedia: struct in C programming language
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack OverflowFind centralized, trusted content and collaborate around the technologies you use most. Q&A for work Connect and share knowledge within a single location that is structured and easy to search. Get early access and see previews of new features. Struct assignment or memcpy? [duplicate]If I want to replicate a structure in another one (in C), what are the pro&con's of : Are they equivalent ? Is there a difference in performance or memory use ?  - 4 Just be careful of memory allocation inside the struct. For example if you have a struct that contains a pointer to a string and you allocate memory for the string. That memory does not get copied. the pointer to the memory gets copied, but not the memory itself. In other word this type of assignment is not a deep copy. Neither is vanilla memcpy for that mater. It can get confusing as to who owns the allocated memory. – Pemdas Commented Mar 21, 2011 at 15:22
- I think this question is more suited to Stackoverflow. – karlphillip Commented Mar 21, 2011 at 16:52
- 2 I think this question was already well answered here: stackoverflow.com/q/4931123/176769 – karlphillip Commented Mar 21, 2011 at 16:54
4 Answers 4The struct1=struct2; notation is not only more concise, but also shorter and leaves more optimization opportunities to the compiler. The semantic meaning of = is an assignment, while memcpy just copies memory. That's a huge difference in readability as well, although memcpy does the same in this case.  - 3 Major problem on s = mystruct between struct s and struct * s. Both work nicely with equals, so you can't see the difference by reading. *s gives you identical, while struct s gives you a copy. When it comes time to deallocate(s), this works fine with the identical struct * s; but it leaks mystruct if you foolishly do only one dealloc with the struct s version. It is also faster to work with pointers. Just keep what you're doing in mind, and then things should be fine. YMMV. – DragonLord Commented Mar 7, 2015 at 2:55
I'm not sure of the performance difference, although I would guess most compilers would use memcpy under the hood. I would prefer the assignment in most cases, it is much easier to read and is much more explicit as to what the purpose is. Imagine you changed the type of either of the structs, the compiler would guide you to what changes were needed, either giving a compiler error or by using an operator= (if one exists). Whereas the second one would blindly do the copy with the possibility of causing a subtle bug.  Check out this conversation about the very same topic: http://bytes.com/topic/c/answers/670947-struct-assignment Basically, there are a lot of disagreements about the corner cases in that thread on what the struct copy would do. It's pretty clear if all the members of a struct are simple values (int, double, etc.). The confusion comes in with what happens with arrays and pointers, and padding bytes. Everything should be pretty clear as to what happens with the memcpy , as that is a verbatim copy of every byte. This includes both absolute memory pointers, relative offsets, etc.  There is no inherent reason why one would be better in performance than the other. Different compilers, and versions of them, may differ, so if you really care, you profile and benchmark and use facts as a basis to decide. A straight assignment is clearer to read. Assignment is a teeny bit riskier to get wrong, so that you assign pointers to structs rather than the structs pointed to. If you fear this, you'll make sure your unit tests cover this. I would not care about this risk. (Similarly, memcpy is risky because you might get the struct size wrong.) - There's nothing risky about memcpy if you use sizeof and the correct struct. I suppose you could get the struct wrong and there would be no error. I think you'd probably get a segmentation fault eventually though. – Michael K Commented Mar 21, 2011 at 17:05
Not the answer you're looking for? Browse other questions tagged c struct variable-assignment memcpy or ask your own question .- The Overflow Blog
- One of the best ways to get value for AI coding tools: generating tests
- The world’s largest open-source business has plans for enhancing LLMs
- Featured on Meta
- User activation: Learnings and opportunities
- Site maintenance - Mon, Sept 16 2024, 21:00 UTC to Tue, Sept 17 2024, 2:00...
- What does a new user need in a homepage experience on Stack Overflow?
- Announcing the new Staging Ground Reviewer Stats Widget
Hot Network Questions- Why is the area covered by 1 steradian (in a sphere) circular in shape?
- How can I analyze the anatomy of a humanoid species to create sounds for their language?
- Proving that a function from one metric space to another is continuous or discontinuous at a point
- Equation of Time (derivation Analemma)
- Taylor Swift - Use of "them" in her text "she fights for the rights and causes I believe need a warrior to champion them"
- Should tiny dimension tables be considered for row or page compression on servers with ample CPU room?
- Was Willy Wonka correct when he accused Charlie of stealing Fizzy Lifting Drinks?
- ASCII 2D landscape
- Is it defamatory to publish nonsense under somebody else's name?
- Concerns with newly installed floor tile
- How to Place a Smooth "Breathing" Above the E with Textgreek?
- Does SpaceX Starship have significant methane emissions?
- Help on PID circuit design
- Navigating career options after a disastrous PhD performance and a disappointed advisor?
- O(nloglogn) Sorting Algorithm?
- "There is a bra for every ket, but there is not a ket for every bra"
- Function with memories of its past life
- How should I email HR after an unpleasant / annoying interview?
- VBA: Efficiently Organise Data with Missing Values to Achieve Minimum Number of Tables
- Proper use of voices in more complicated melodies
- Is it feasible to create an online platform to effectively teach college-level math (abstract algebra, real analysis, etc.)?
- What would be an appropriate translation of Solitude?
- How to decrease by 1 integers in an expl3's clist?
- Non-existence of power divided structure on a maximal ideal of truncated polynomial rings (example from Koblitz)
 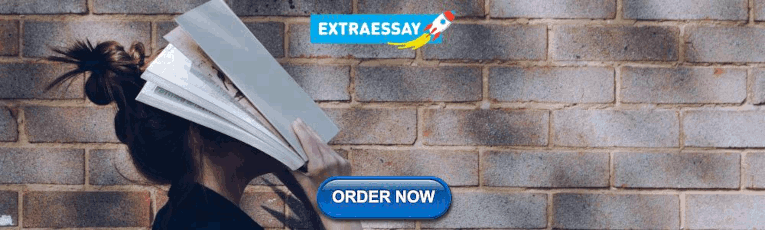 |
IMAGES
VIDEO
COMMENTS
4. Yes, you can assign one instance of a struct to another using a simple assignment statement. In the case of non-pointer or non pointer containing struct members, assignment means copy. In the case of pointer struct members, assignment means pointer will point to the same address of the other pointer.
15.13 Structure Assignment. Assignment operating on a structure type copies the structure. The left and right operands must have the same type. Here is an example: Notionally, assignment on a structure type works by copying each of the fields. Thus, if any of the fields has the const qualifier, that structure type does not allow assignment:
The structure in C is a user-defined data type that can be used to group items of possibly different types into a single type. The struct keyword is used to define the structure in the C programming language. The items in the structure are called its member and they can be of any valid data type. Additionally, the values of a structure are stored in contiguous memory locations.
To access the structure, you must create a variable of it. Use the struct keyword inside the main() method, followed by the name of the structure and then the name of the structure variable: Create a struct variable with the name "s1": struct myStructure {. int myNum; char myLetter; }; int main () {. struct myStructure s1;
Example of Nested Structure in C Programming. Let's say we have two structure like this: The second structure stu_data has stu_address as a data member. Here, stu_data is called outer structure or parent structure and stu_address is called inner structure or child structure. Structure 1: stu_address. struct stu_address.
In this tutorial, you'll learn about struct types in C Programming. You will learn to define and use structures with the help of examples. In C programming, a struct (or structure) is a collection of variables (can be of different types) under a single name. ... and we cannot use the assignment operator = with it after we have declared the ...
Abdelghani Bellaachia, CSCI 1121 Page: 6 4. Manipulating Structure Types How to access a field in a structure: o Use the direct component selection operator, which is a period. o The direct component selection operator has the highest priority in the operator precedence. o Examples: person p1; p1.name; p1.age; Structure assignment: o The copy of an entire structure can be easily done
C++ code defining a function named read that returns a structure. The program defines and initializes the structure object, temp, in local or function scope with a series of console read operations and returns it as the function return value. The assignment operator saves the returned structure in s3.
structattr-spec-seq (optional)name. (2) 1) Struct definition: introduces the new type struct name and defines its meaning. 2) If used on a line of its own, as in structname;, declares but doesn't define the struct name (see forward declaration below). In other contexts, names the previously-declared struct, and attr-spec-seq is not allowed.
Structure may contain different data types such as char, integer, float and group them together to create a user-defined data type. ¶Declaring Structure in C. To declare a structure, we use the keyword struct followed by an identifier as the structure name. Inside the curly braces we list the member variables with their respective data types.
Notes for You:: C Structure Assignment.Example Code:#include <stdio.h>int main(){ struct Date { int day; int month; int year...
Arrow operator (->) in C. Since structure is a user defined type and you can have pointers to any type. Hence, you may also create pointers to structure. In C language it is illegal to access a structure member from a pointer to structure variable using dot operator. We use arrow operator -> to access structure member from pointer to structure ...
printf("weight: %f", personPtr->weight); return 0; } Run Code. In this example, the address of person1 is stored in the personPtr pointer using personPtr = &person1;. Now, you can access the members of person1 using the personPtr pointer. By the way, personPtr->age is equivalent to (*personPtr).age. personPtr->weight is equivalent to ...
Note also that although in C memcpy and structure assignment are usually equivalent, in C++ memcpy and structure assignment are not equivalent. In general C++ it's best to avoid memcpying structures, as structure assignment can, and often is, overloaded to do additional things such as deep copies or reference count management.
1. By separate nested structure: In this method, the two structures are created, but the dependent structure (Employee) should be used inside the main structure (Organisation) as a member. Below is the C program to implement the approach: C. #include <stdio.h>. #include <string.h>. struct Employee.
Structures in C. A structure in C is a derived or user-defined data type. We use the keyword struct to define a custom data type that groups together the elements of different types. The difference between an array and a structure is that an array is a homogenous collection of similar types, whereas a structure can have elements of different types stored adjacently and identified by a name.
C struct Examples. Store information of a student using structure. Add two distances (in inch-feet) Add two complex numbers by passing structures to a function. Calculate the difference between two time periods. Store information of 10 students using structures. Store information of n students using structures. Share on:
If structure has pointer or array member, please consider the pointer alias problem, it will lead dangling pointer once incorrect use. Better way is implement structure assignment function in C, and overload the operator= function in C++. Reference: stackoverflow.com: structure assignment or memcpy; stackoverflow.com: assign one struct to ...
Interesting that the accepted (and heavily upvoted) answer doesn't actually answer the question, even as originally posted. Designated initializers don't address the OP's problem, which is to split the declaration from the initialization. For pre-1999 C, the only real solution is to assign to each member; for C99 and later, a compound literal, as in CesarB's answer, is the solution.
Move Assignment (C++11) If you want to know why? It is to maintain backward compatibility with C (because C structs are copyable using = and in declaration). But it also makes writing simple classes easier. ... // zero-initialized // some_struct c; // value-initialized // some_struct d = some_struct(); // zero-initialized // Note: Just because ...
The struct1=struct2; notation is not only more concise, but also shorter and leaves more optimization opportunities to the compiler. The semantic meaning of = is an assignment, while memcpy just copies memory. That's a huge difference in readability as well, although memcpy does the same in this case. Use =.