Go Tutorial
Go exercises, go assignment operators, assignment operators.
Assignment operators are used to assign values to variables.
In the example below, we use the assignment operator ( = ) to assign the value 10 to a variable called x :
The addition assignment operator ( += ) adds a value to a variable:
A list of all assignment operators:
Operator | Example | Same As | Try it |
---|---|---|---|
= | x = 5 | x = 5 | |
+= | x += 3 | x = x + 3 | |
-= | x -= 3 | x = x - 3 | |
*= | x *= 3 | x = x * 3 | |
/= | x /= 3 | x = x / 3 | |
%= | x %= 3 | x = x % 3 | |
&= | x &= 3 | x = x & 3 | |
|= | x |= 3 | x = x | 3 | |
^= | x ^= 3 | x = x ^ 3 | |
>>= | x >>= 3 | x = x >> 3 | |
<<= | x <<= 3 | x = x << 3 |

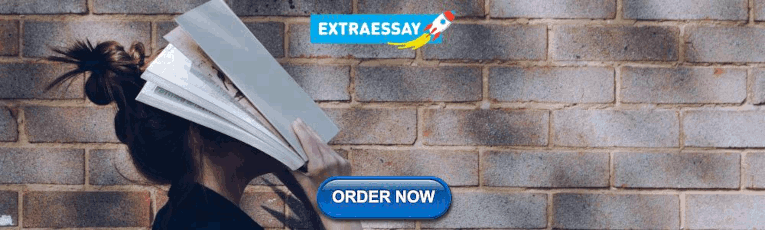
COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
Difference between := and = operators in Go
What is the difference between the = and := operators, and what are the use cases for them? They both seem to be for an assignment?
- 3 Also see this : Go Variables Visual Guide . I wrote an article about it. – Inanc Gumus Commented Nov 20, 2017 at 13:39
- 1 The semantics... – JustDave Commented Jan 27, 2018 at 15:27
- If you want to use a specific type x := uint32(123) works for example. It must be obvious for most people but I had to think a few minutes ;-) – Kenji Noguchi Commented Dec 9, 2019 at 23:35
- I highly recommend starting with the Tour of Go: tour.golang.org/basics/9 – Haris Osmanagić Commented Nov 1, 2021 at 14:07
8 Answers 8
In Go, := is for declaration + assignment, whereas = is for assignment only.
For example, var foo int = 10 is the same as foo := 10 .
- 9 Is there a use case for = as opposed to := ? Should you just always use := ? – Kenny Worden Commented Jul 27, 2017 at 15:45
- 13 @KennethWorden Go won't let you use := to assign to a variable that has already been declared, unless you are assigning to multiple variables at once, and at least one of those variables is new. – Kenny Bania Commented Aug 30, 2017 at 18:36
- 15 the int is not required, var foo = 10 is the same as foo := 10 – Gary Lyn Commented Nov 21, 2017 at 14:04
- 5 @KennyWorden, yes. You can't use := outside a function. – karuhanga Commented Aug 10, 2019 at 20:15
- 2 I believe the := is used for type inference as well, you can use it like ` i := 1 ` as opposed to ` var i int = 1 ` – nulltron Commented Feb 12, 2022 at 6:05
- Declare a variable, use: := .
- Change a variable’s value, use: = .
There are some rules and the TLDR is not always true.
As others have explained already, := is for both declaration, assignment, and also for redeclaration; and it guesses ( infers ) the variable's type automatically.
For example, foo := 32 is a short-hand form of:
/ Here are the rules: /
★ 1st Rule:
You can't use := outside of funcs . It's because, outside a func, a statement should start with a keyword.
★ 2nd Rule:
You can't use them twice ( in the same scope ):
Because, := introduces "a new variable" , hence using it twice does not redeclare a second variable, so it's illegal.
★ 3rd Rule:
You can use them for multi-variable declarations and assignments:
★ 4th Rule (Redeclaration):
You can use them twice in "multi-variable" declarations, if one of the variables is new :
This is legal, because, you're not declaring all the variables, you're just reassigning new values to the existing variables, and declaring new variables at the same time. This is called redeclaration .
★ 5th Rule:
You can use the short declaration to declare a variable in a newer scope even if that variable is already declared with the same name before:
Here, foo := 42 is legal, because, it declares foo in some() func's scope. foo = 314 is legal, because, it just assigns a new value to foo .
★ 6th Rule:
You can declare the same name in short statement blocks like: if , for , switch :
Because, foo in if foo := ... , only belongs to that if clause and it's in a different scope.
References:
Short Variable Declaration Rules
A Visual Guide to Go Variables
Only = is the assignment operator .
:= is a part of the syntax of the short variable declaration clause. 👉 There are some rules though. See this other answer for more details.

- 1 Looks like := is listed as an operator here golang.org/ref/spec#Operators_and_punctuation , so I'm not sure I agree that " := is actually not an operator" – Powers Commented Jun 25, 2018 at 2:57
:= is a short-hand for declaration.
a will be declared as an int and initialized with value 10 where as b will be declared as a string and initialized with value gopher .
Their equivalents using = would be
= is assignment operator. It is used the same way you would use it in any other language.
You can omit the type when you declare the variable and an initializer is present ( http://tour.golang.org/#11 ).
- «= is assignment operator. It is used the same way you would use it in any other language.» Except in Ada where = is only for comparison and := is for assignment... – Alexis Wilke Commented Jul 16, 2019 at 6:26
The := means declare and assign while the = means to simply assign.
:= declares and assigns, = just assigns
It's useful when you don't want to fill up your code with type or struct declarations.
from the reference doc : ( tour.golang.org )
Inside a function , the := short assignment statement can be used in place of a var declaration with implicit type.
Outside a function , every construct begins with a keyword (var, func, and so on) and the := construct is not available.
In Go := is for declaration and assignment also whereas = is only for the Assignment
for example:

Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged go or ask your own question .
- The Overflow Blog
- From PHP to JavaScript to Kubernetes: how one backend engineer evolved over time
- Featured on Meta
- We've made changes to our Terms of Service & Privacy Policy - July 2024
- Bringing clarity to status tag usage on meta sites
- Feedback requested: How do you use tag hover descriptions for curating and do...
- What does a new user need in a homepage experience on Stack Overflow?
Hot Network Questions
- Definition of basis in infinite dimensional vector space
- What's the origin of the colloquial "peachy", "simply peachy", and "just peachy"?
- What does "close" mean in the phrase: "close a tooth pick between the jamb and the door"?
- How can you trust a forensic scientist to have maintained the chain of custody?
- Show that a sinusoidal is an eigenfunction of an LTI system
- Opamp Input Noise Source?
- A short story about a SF author telling his friends, as a joke, a harebrained SF story, and what follows
- Unable to locate package epstopdf
- When was this photo taken?
- In theory, could an object like 'Oumuamua have been captured by a three-body interaction with the sun and planets?
- Will this be the first time that there are more people aboad the ISS than seats in docked spacecraft?
- If Miles doesn’t consider Peter’s actions as hacking, then what does he think Peter is doing to the computer?
- Comparing force-carrying particles
- Meaning of “ ’thwart” in a 19th century poem
- Is the error in translation of Genesis 19:5 deliberate?
- Reduce String Length With Thread Safety & Concurrency
- Can the subjunctive mood be combined with ‘Be to+infinitive’?
- Do mini-humans need a "real" Saturn V to reach the moon?
- Using ExplSyntax with tasks to create a custom \item command - Making \item backwards compatible
- Seth and Cain take turns picking numbers from 1 to 50. Who wins?
- Subdomain takeover with A record
- If more collisions happen with more resistance, why less heat is generated?
- Aligning columns with multicolumn header
- Which game is "that 651"?
- Trending Now
- Foundational Courses
- Data Science
- Practice Problem
- Machine Learning
- System Design
- DevOps Tutorial
Go Operators
Operators are the foundation of any programming language. Thus the functionality of the Go language is incomplete without the use of operators. Operators allow us to perform different kinds of operations on operands. In the Go language , operators Can be categorized based on their different functionality:
Arithmetic Operators
Relational operators, logical operators, bitwise operators, assignment operators, misc operators.
These are used to perform arithmetic/mathematical operations on operands in Go language:
- Addition: The ‘+’ operator adds two operands. For example, x+y.
- Subtraction: The ‘-‘ operator subtracts two operands. For example, x-y.
- Multiplication: The ‘*’ operator multiplies two operands. For example, x*y.
- Division: The ‘/’ operator divides the first operand by the second. For example, x/y.
- Modulus: The ‘%’ operator returns the remainder when the first operand is divided by the second. For example, x%y.
Note: -, +, !, &, *, <-, and ^ are also known as unary operators and the precedence of unary operators is higher. ++ and — operators are from statements they are not expressions, so they are out from the operator hierarchy.
Example:
Output:
Relational operators are used for the comparison of two values. Let’s see them one by one:
- ‘=='(Equal To) operator checks whether the two given operands are equal or not. If so, it returns true. Otherwise, it returns false. For example, 5==5 will return true.
- ‘!='(Not Equal To) operator checks whether the two given operands are equal or not. If not, it returns true. Otherwise, it returns false. It is the exact boolean complement of the ‘==’ operator. For example, 5!=5 will return false.
- ‘>'(Greater Than) operator checks whether the first operand is greater than the second operand. If so, it returns true. Otherwise, it returns false. For example, 6>5 will return true.
- ‘<‘(Less Than) operator checks whether the first operand is lesser than the second operand. If so, it returns true. Otherwise, it returns false. For example, 6<5 will return false.
- ‘>='(Greater Than Equal To) operator checks whether the first operand is greater than or equal to the second operand. If so, it returns true. Otherwise, it returns false. For example, 5>=5 will return true.
- ‘<='(Less Than Equal To) operator checks whether the first operand is lesser than or equal to the second operand. If so, it returns true. Otherwise, it returns false. For example, 5<=5 will also return true.
They are used to combine two or more conditions/constraints or to complement the evaluation of the original condition in consideration.
- Logical AND: The ‘&&’ operator returns true when both the conditions in consideration are satisfied. Otherwise it returns false. For example, a && b returns true when both a and b are true (i.e. non-zero).
- Logical OR: The ‘||’ operator returns true when one (or both) of the conditions in consideration is satisfied. Otherwise it returns false. For example, a || b returns true if one of a or b is true (i.e. non-zero). Of course, it returns true when both a and b are true.
- Logical NOT: The ‘!’ operator returns true the condition in consideration is not satisfied. Otherwise it returns false. For example, !a returns true if a is false, i.e. when a=0.
In Go language, there are 6 bitwise operators which work at bit level or used to perform bit by bit operations. Following are the bitwise operators :
- & (bitwise AND): Takes two numbers as operands and does AND on every bit of two numbers. The result of AND is 1 only if both bits are 1.
- | (bitwise OR): Takes two numbers as operands and does OR on every bit of two numbers. The result of OR is 1 any of the two bits is 1.
- ^ (bitwise XOR): Takes two numbers as operands and does XOR on every bit of two numbers. The result of XOR is 1 if the two bits are different.
- << (left shift): Takes two numbers, left shifts the bits of the first operand, the second operand decides the number of places to shift.
- >> (right shift): Takes two numbers, right shifts the bits of the first operand, the second operand decides the number of places to shift.
- &^ (AND NOT): This is a bit clear operator.
Assignment operators are used to assigning a value to a variable. The left side operand of the assignment operator is a variable and right side operand of the assignment operator is a value. The value on the right side must be of the same data-type of the variable on the left side otherwise the compiler will raise an error. Different types of assignment operators are shown below:
- “=”(Simple Assignment): This is the simplest assignment operator. This operator is used to assign the value on the right to the variable on the left.
- “+=”(Add Assignment): This operator is a combination of ‘+’ and ‘=’ operators. This operator first adds the current value of the variable on left to the value on the right and then assigns the result to the variable on the left.
- “-=”(Subtract Assignment): This operator is a combination of ‘-‘ and ‘=’ operators. This operator first subtracts the current value of the variable on left from the value on the right and then assigns the result to the variable on the left.
- “*=”(Multiply Assignment): This operator is a combination of ‘*’ and ‘=’ operators. This operator first multiplies the current value of the variable on left to the value on the right and then assigns the result to the variable on the left.
- “/=”(Division Assignment): This operator is a combination of ‘/’ and ‘=’ operators. This operator first divides the current value of the variable on left by the value on the right and then assigns the result to the variable on the left.
- “%=”(Modulus Assignment): This operator is a combination of ‘%’ and ‘=’ operators. This operator first modulo the current value of the variable on left by the value on the right and then assigns the result to the variable on the left.
- “&=”(Bitwise AND Assignment): This operator is a combination of ‘&’ and ‘=’ operators. This operator first “Bitwise AND” the current value of the variable on the left by the value on the right and then assigns the result to the variable on the left.
- “^=”(Bitwise Exclusive OR): This operator is a combination of ‘^’ and ‘=’ operators. This operator first “Bitwise Exclusive OR” the current value of the variable on left by the value on the right and then assigns the result to the variable on the left.
- “|=”(Bitwise Inclusive OR): This operator is a combination of ‘|’ and ‘=’ operators. This operator first “Bitwise Inclusive OR” the current value of the variable on left by the value on the right and then assigns the result to the variable on the left.
- “<<=”(Left shift AND assignment operator): This operator is a combination of ‘<<’ and ‘=’ operators. This operator first “Left shift AND” the current value of the variable on left by the value on the right and then assigns the result to the variable on the left.
- “>>=”(Right shift AND assignment operator): This operator is a combination of ‘>>’ and ‘=’ operators. This operator first “Right shift AND” the current value of the variable on left by the value on the right and then assigns the result to the variable on the left.
- &: This operator returns the address of the variable.
- *: This operator provides pointer to a variable.
- <-: The name of this operator is receive. It is used to receive a value from the channel.
Please Login to comment...
Similar reads.
- How to Get a Free SSL Certificate
- Best SSL Certificates Provider in India
- Elon Musk's xAI releases Grok-2 AI assistant
- What is OpenAI SearchGPT? How it works and How to Get it?
- Content Improvement League 2024: From Good To A Great Article
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Popular Tutorials
Popular examples, learn python interactively, go introduction.
- Golang Getting Started
- Go Variables
- Go Data Types
- Go Print Statement
- Go Take Input
- Go Comments
Go Operators
- Go Type Casting
Go Flow Control
- Go Boolean Expression
- Go if...else
- Go for Loop
- Go while Loop
- Go break and continue
Go Data Structures
- Go Functions
- Go Variable Scope
- Go Recursion
- Go Anonymous Function
- Go Packages
Go Pointers & Interface
Go Pointers
- Go Pointers and Functions
- Go Pointers to Struct
- Go Interface
- Go Empty Interface
- Go Type Assertions
Go Additional Topics
Go defer, panic, and recover
Go Tutorials
Go Booleans (Relational and Logical Operators)
- Go Pointers to Structs
In Computer Programming, an operator is a symbol that performs operations on a value or a variable.
For example, + is an operator that is used to add two numbers.
Go programming provides wide range of operators that are categorized into following major categories:
- Arithmetic operators
- Assignment operator
- Relational operators
- Logical operators
- Arithmetic Operator
We use arithmetic operators to perform arithmetic operations like addition, subtraction, multiplication, and division.
Here's a list of various arithmetic operators available in Go.
Operators | Example |
---|---|
(Addition) | |
(Subtraction) | |
(Multiplication) | |
(Division) | |
(Modulo Division) |
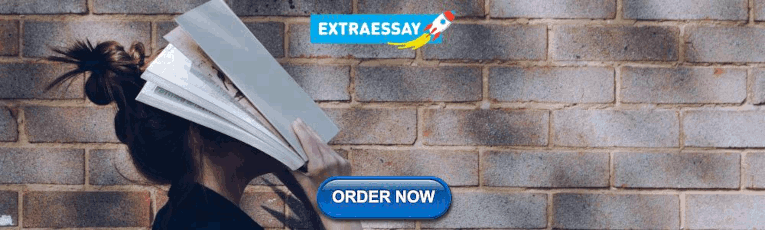
Example 1: Addition, Subtraction and Multiplication Operators
Example 2: golang division operator.
In the above example, we have used the / operator to divide two numbers: 11 and 4 . Here, we get the output 2 .
However, in normal calculation, 11 / 4 gives 2.75 . This is because when we use the / operator with integer values, we get the quotients instead of the actual result.
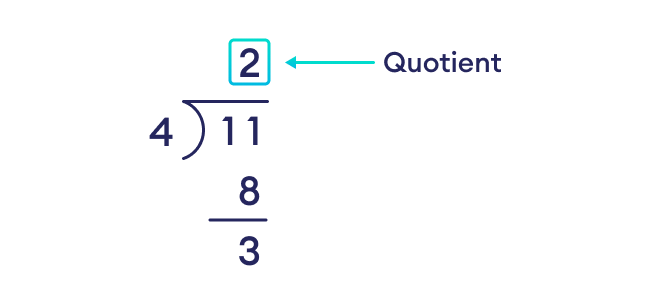
If we want the actual result we should always use the / operator with floating point numbers. For example,
Here, we get the actual result after division.
Example 3: Modulus Operator in Go
In the above example, we have used the modulo operator with numbers: 11 and 4 . Here, we get the result 3 .
This is because in programming, the modulo operator always returns the remainder after division.
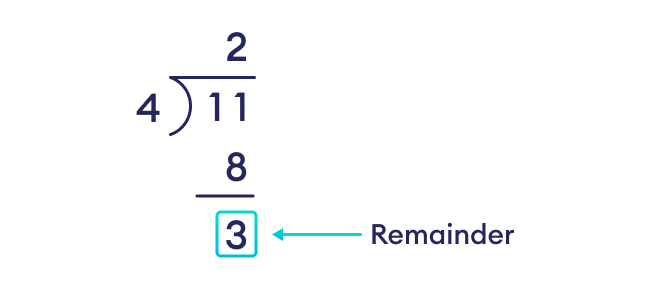
Note: The modulo operator is always used with integer values.
- Increment and Decrement Operator in Go
In Golang, we use ++ (increment) and -- (decrement) operators to increase and decrease the value of a variable by 1 respectively. For example,
In the above example,
- num++ - increases the value of num by 1 , from 5 to 6
- num-- - decreases the value of num by 1 , from 5 to 4
Note: We have used ++ and -- as prefixes (before variable). However, we can also use them as postfixes ( num++ and num-- ).
There is a slight difference between using increment and decrement operators as prefixes and postfixes. To learn the difference, visit Increment and Decrement Operator as Prefix and Postfix .
- Go Assignment Operators
We use the assignment operator to assign values to a variable. For example,
Here, the = operator assigns the value on right ( 34 ) to the variable on left ( number ).
Example: Assignment Operator in Go
In the above example, we have used the assignment operator to assign the value of the num variable to the result variable.
- Compound Assignment Operators
In Go, we can also use an assignment operator together with an arithmetic operator. For example,
Here, += is additional assignment operator. It first adds 6 to the value of number ( 2 ) and assigns the final result ( 8 ) to number .
Here's a list of various compound assignment operators available in Golang.
Operator | Example | Same as |
---|---|---|
(addition assignment) | ||
(subtraction assignment) | ||
(multiplication assignment) | ||
(division assignment) | ||
(modulo assignment) |
- Relational Operators in Golang
We use the relational operators to compare two values or variables. For example,
Here, == is a relational operator that checks if 5 is equal to 6 .
A relational operator returns
- true if the comparison between two values is correct
- false if the comparison is wrong
Here's a list of various relational operators available in Go:
Operator | Example | Descriptions |
---|---|---|
(equal to) | returns if and are equal | |
(not equal to) | returns if and are not equal | |
(greater than) | returns if is greater than | |
(less than) | returns if is less than | |
(greater than or equal to) | returns if is either greater than or equal to | |
(less than or equal to) | returns is is either less than or equal to |
To learn more, visit Go relational operators .
- Logical Operators in Go
We use the logical operators to perform logical operations. A logical operator returns either true or false depending upon the conditions.
Operator | Description | Example |
---|---|---|
(Logical AND) | returns if both expressions and are | |
(Logical OR) | returns if any one of the expressions is . | |
(Logical NOT) | returns if is and returns if is . |
To learn more, visit Go logical operators .
More on Go Operators
The right shift operator shifts all bits towards the right by a certain number of specified bits.
Suppose we want to right shift a number 212 by some bits then,
For example,
The left shift operator shifts all bits towards the left by a certain number of specified bits. The bit positions that have been vacated by the left shift operator are filled with 0 .
Suppose we want to left shift a number 212 by some bits then,
In Go, & is the address operator that is used for pointers. It holds the memory address of a variable. For example,
Here, we have used the * operator to declare the pointer variable. To learn more, visit Go Pointers .
In Go, * is the dereferencing operator used to declare a pointer variable. A pointer variable stores the memory address. For example,
In Go, we use the concept called operator precedence which determines which operator is executed first if multiple operators are used together. For example,
Here, the / operator is executed first followed by the * operator. The + and - operators are respectively executed at last.
This is because operators with the higher precedence are executed first and operators with lower precedence are executed last.
Table of Contents
- Introduction
- Example: Addition, Subtraction and Multiplication
- Golang Division Operator
Sorry about that.
Related Tutorials
Programming
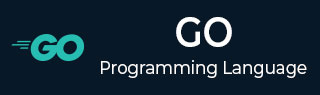
- Go Tutorial
- Go - Overview
- Go - Environment Setup
- Go - Program Structure
- Go - Basic Syntax
- Go - Data Types
- Go - Variables
- Go - Constants
- Go - Operators
- Go - Decision Making
- Go - Functions
- Go - Scope Rules
- Go - Strings
- Go - Arrays
- Go - Pointers
- Go - Structures
- Go - Recursion
- Go - Type Casting
- Go - Interfaces
- Go - Error Handling
- Go Useful Resources
- Go - Questions and Answers
- Go - Quick Guide
- Go - Useful Resources
- Go - Discussion
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
Go - Assignment Operators
The following table lists all the assignment operators supported by Go language −
Operator | Description | Example |
---|---|---|
= | Simple assignment operator, Assigns values from right side operands to left side operand | C = A + B will assign value of A + B into C |
+= | Add AND assignment operator, It adds right operand to the left operand and assign the result to left operand | C += A is equivalent to C = C + A |
-= | Subtract AND assignment operator, It subtracts right operand from the left operand and assign the result to left operand | C -= A is equivalent to C = C - A |
*= | Multiply AND assignment operator, It multiplies right operand with the left operand and assign the result to left operand | C *= A is equivalent to C = C * A |
/= | Divide AND assignment operator, It divides left operand with the right operand and assign the result to left operand | C /= A is equivalent to C = C / A |
%= | Modulus AND assignment operator, It takes modulus using two operands and assign the result to left operand | C %= A is equivalent to C = C % A |
<<= | Left shift AND assignment operator | C <<= 2 is same as C = C << 2 |
>>= | Right shift AND assignment operator | C >>= 2 is same as C = C >> 2 |
&= | Bitwise AND assignment operator | C &= 2 is same as C = C & 2 |
^= | bitwise exclusive OR and assignment operator | C ^= 2 is same as C = C ^ 2 |
|= | bitwise inclusive OR and assignment operator | C |= 2 is same as C = C | 2 |
Try the following example to understand all the assignment operators available in Go programming language −
When you compile and execute the above program it produces the following result −
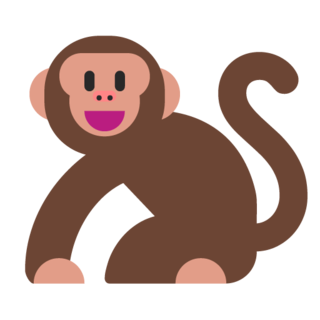
Go Assignment Operators
What are assignment operators.
Assignment operators are used to assign variables to values.
The Assignment Operator ( = )
The equal sign that we are all familiar with is called the assignment operator, because it is the most important out of all the assignment operators. All the other assignment operators are built off of it. For example, the below code assigns the variable a the value of 3, the constant pi the value of 3.14, and the variable website the value of Learnmonkey:
Notice that we can use the assignment operator to make constants and variables. We can also use the assignment operator to set variables (not constants) once they are already created:
Other Assignment Operators
The other assignment operators were created so that as developers, our lives would be easier. They are all equivalent to using the assignment operator in some way:
- Introduction to Go
- Environment Setup
- Your First Go Program
- More on Printing
Go operators
last modified April 11, 2024
In this article we cover Go operators. We show how to use operators to create expressions.
An operator is a special symbol which indicates a certain process is carried out. Operators in programming languages are taken from mathematics. Programmers work with data. The operators are used to process data. An operand is one of the inputs (arguments) of an operator.
Expressions are constructed from operands and operators. The operators of an expression indicate which operations to apply to the operands. The order of evaluation of operators in an expression is determined by the precedence and associativity of the operators.
An operator usually has one or two operands. Those operators that work with only one operand are called unary operators . Those who work with two operands are called binary operators .
Certain operators may be used in different contexts. For instance the + operator can be used in different cases: it adds numbers, concatenates strings, or indicates the sign of a number. We say that the operator is overloaded .
Go sign operators
There are two sign operators: + and - . They are used to indicate or change the sign of a value.
The + and - signs indicate the sign of a value. The plus sign can be used to signal that we have a positive number. It can be omitted and it is in most cases done so.
The minus sign changes the sign of a value.
Go assignment operator
The assignment operator = assigns a value to a variable. A variable is a placeholder for a value. In mathematics, the = operator has a different meaning. In an equation, the = operator is an equality operator. The left side of the equation is equal to the right one.
This expression does not make sense in mathematics, but it is legal in programming. The expression adds 1 to the x variable. The right side is equal to 2 and 2 is assigned to x .
This code line leads to a syntax error. We cannot assign a value to a literal.
Go increment and decrement operators
In the above example, we demonstrate the usage of both operators.
We initiate the x variable to 6. Then we increment x two times. Now the variable equals to 8.
Go compound assignment operators
The compound assignment operators consist of two operators. They are shorthand operators.
In the code example, we use two compound operators.
Using a += compound operator, we add 5 to the a variable. The statement is equal to a = a + 5 .
Go arithmetic operators
Symbol | Name |
---|---|
Addition | |
Subtraction | |
Multiplication | |
/ | Division |
Remainder |
The % operator is called the remainder or the modulo operator. It finds the remainder of division of one number by another. For example, 9 % 4 , 9 modulo 4 is 1, because 4 goes into 9 twice with a remainder of 1.
If one of the values is a double or a float, we perform a floating point division. In our case, the second operand is a double so the result is a double.
Go Boolean operators
Symbol | Name |
---|---|
logical and | |
logical or | |
negation |
Boolean operators are also called logical.
Relational operators always result in a boolean value. These two lines print false and true.
The body of the if statement is executed only if the condition inside the parentheses is met. The y > x returns true, so the message "y is greater than x" is printed to the terminal.
If one of the sides of the operator is true, the outcome of the operation is true.
Three of four expressions result in true.
Go comparison operators
Symbol | Meaning |
---|---|
less than | |
less than or equal to | |
greater than | |
greater than or equal to | |
equal to | |
not equal to |
comparison operators are also called relational operators.
Go bitwise operators
Decimal numbers are natural to humans. Binary numbers are native to computers. Binary, octal, decimal, or hexadecimal symbols are only notations of the same number. Bitwise operators work with bits of a binary number.
Symbol | Meaning |
---|---|
bitwise exclusive or | |
bitwise and | |
bitwise or | |
bit clear (and not) | |
left shift | |
right shift |
The bitwise and operator performs bit-by-bit comparison between two numbers. The result for a bit position is 1 only if both corresponding bits in the operands are 1.
The first number is a binary notation of 6, the second is 3, and the result is 2.
The bitwise or operator performs bit-by-bit comparison between two numbers. The result for a bit position is 1 if either of the corresponding bits in the operands is 1.
The result is 00110 or decimal 7.
The bitwise exclusive or operator performs bit-by-bit comparison between two numbers. The result for a bit position is 1 if one or the other (but not both) of the corresponding bits in the operands is 1.
The result is 00101 or decimal 5.
Go pointer operators
In the code example, we demonstrate the two operators.
We get the address of the count variable; we create a pointer to the variable.
Again, via pointer dereference, we print the value to which the pointer refers.
Go channel operator
The example presents the channel operator.
Go operator precedence
The operator precedence tells us which operators are evaluated first. The precedence level is necessary to avoid ambiguity in expressions.
What is the outcome of the following expression, 28 or 40?
Like in mathematics, the multiplication operator has a higher precedence than addition operator. So the outcome is 28.
To change the order of evaluation, we can use parentheses. Expressions inside parentheses are always evaluated first. The result of the above expression is 40.
The evaluation of the expression can be altered by using round brackets. In this case, the 3 + 5 is evaluated and later the value is multiplied by 5. This line prints 40.
In this case, the negation operator has a higher precedence than the bitwise or. First, the initial true value is negated to false, then the | operator combines false and true, which gives true in the end.
Associativity rule
What is the outcome of this expression, 9 or 1? The multiplication, deletion, and the modulo operator are left to right associated. So the expression is evaluated this way: (9 / 3) * 3 and the result is 9.
Arithmetic, boolean and relational operators are left to right associated. The ternary operator, increment, decrement, unary plus and minus, negation, bitwise not, type cast, object creation operators are right to left associated.
The compound assignment operators are right to left associated. We might expect the result to be 1. But the actual result is 0. Because of the associativity. The expression on the right is evaluated first and then the compound assignment operator is applied.
In this article we have covered Go operators.
List all Go tutorials .
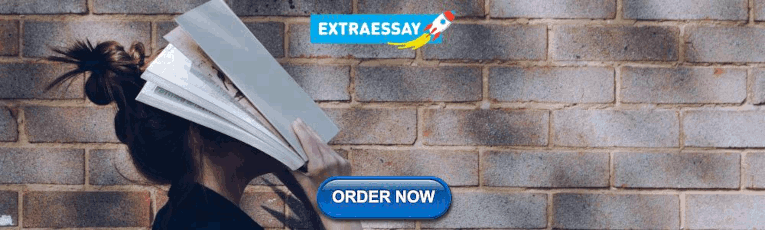
IMAGES
COMMENTS
Lately I was playing with google's new programming language Go and was wondering why the assignment operator := has a colon in front of the equal sign =. Is there a particular reason why the authors of the language wanted to use name := "John" instead of name = "John" ?
Assignment Operators. Assignment operators are used to assign values to variables. In the example below, we use the assignment operator ( =) to assign the value 10 to a variable called x: Example. package main. import ("fmt") func main () { var x = 10. fmt.Println(x) } Try it Yourself »
Strings can be concatenated using the + operator or the += assignment operator: s := "hi" + string(c) s += " and good bye" String addition creates a new string by concatenating the operands.
In Go, := is for declaration + assignment, whereas = is for assignment only. For example, var foo int = 10 is the same as foo := 10.
Different types of assignment operators are shown below: “=” (Simple Assignment): This is the simplest assignment operator. This operator is used to assign the value on the right to the variable on the left. “+=” (Add Assignment): This operator is a combination of ‘+’ and ‘=’ operators.
Go Assignment Operators. We use the assignment operator to assign values to a variable. For example, var number = 34. Here, the = operator assigns the value on right (34) to the variable on left (number).
The following table lists all the assignment operators supported by Go language −. Example. Try the following example to understand all the assignment operators available in Go programming language −. Live Demo. package main. import "fmt" . func main() { var a int = 21 var c int . c = a.
Inside a function, the := short assignment statement can be used in place of a var declaration with implicit type. Outside a function, every statement begins with a keyword ( var , func , and so on) and so the := construct is not available.
Assignment operators are used to assign variables to values. The Assignment Operator ( = ) The equal sign that we are all familiar with is called the assignment operator, because it is the most important out of all the assignment operators.
Go assignment operator. The assignment operator = assigns a value to a variable. A variable is a placeholder for a value. In mathematics, the = operator has a different meaning. In an equation, the = operator is an equality operator. The left side of the equation is equal to the right one. var x = 1.